The purpose
This displays multiple scenes side-by-side in Phaser3.
mainScene
(800×600, but confined to 600×600 on the left) and SecondScene
(200×600 on the right) will be displayed together.
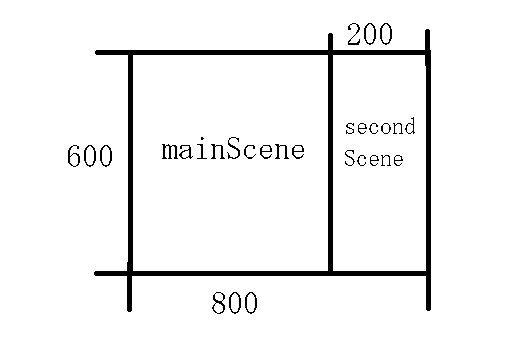
Implementation
Prepare
Create mainScene
The MainScene is implemented as follows:
It is 800×600 pixels in size and filled with a translucent red rectangle. (A gray background is used for clarity.)
class mainScene extends Phaser.Scene {
create ()
{
this.add.rectangle(0, 0, 800, 600, 0xff0000, 0.5).setOrigin(0);
}
}
It will be displayed as follows
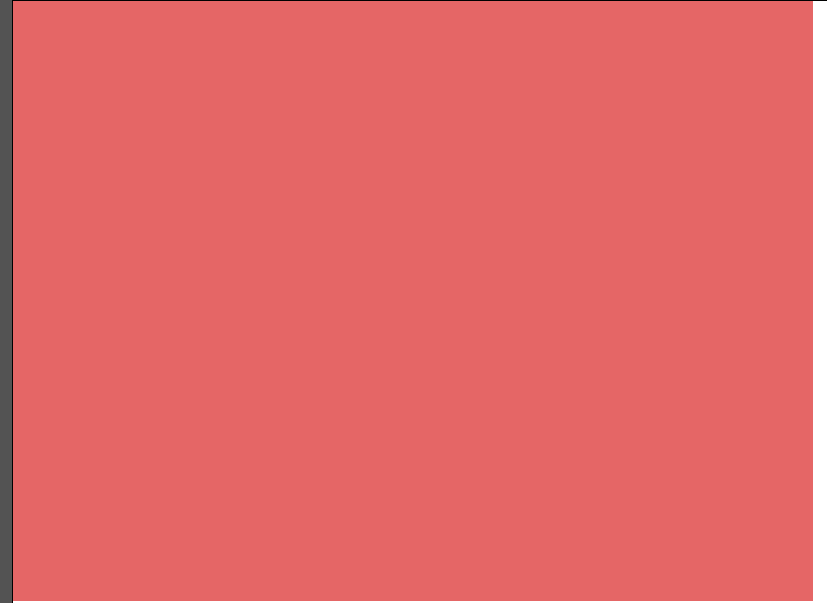
create secondScene
The second scene was created as follows:
It’s filled with a translucent green rectangle.
class secondScene extends Phaser.Scene {
create ()
{
this.add.rectangle(0, 0, 200, 600, 0x00ff00, 0.5).setOrigin(0);
}
}
Limiting the display area
We constrain the display area of mainScene to 600×600 pixels using cameras.main.setSize
.
class mainScene extends Phaser.Scene {
create ()
{
this.cameras.main.setSize(600,600);//追加
this.add.rectangle(0, 0, 800, 600, 0xff0000, 0.5).setOrigin(0);
}
}
The following is displayed. While the rectangle is specified as 800 x 600, it is observed that only 600 pixels in width are actually rendered.
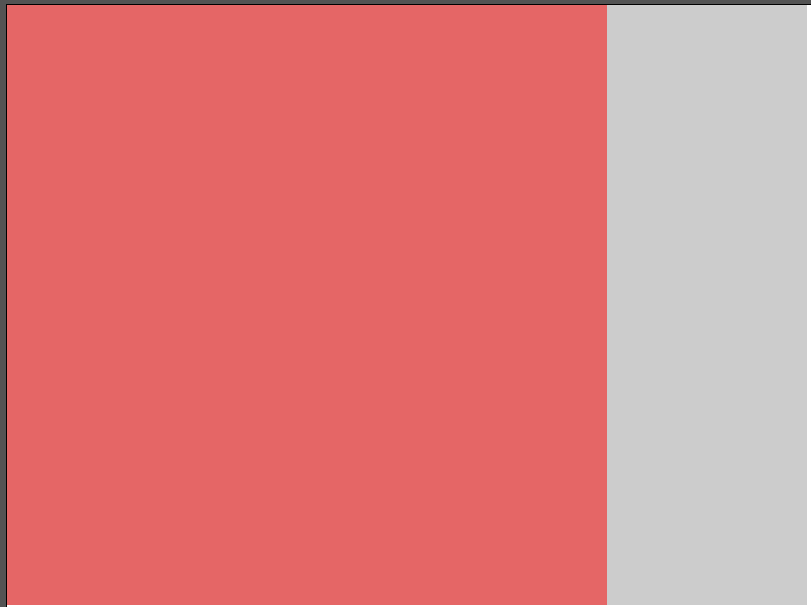
call secondScene
Add secondScene
to mainScene
using this.scene.add
.
The first argument is a name; any unique string will do.
The second argument is the class name.
The third argument is true. (If set to false, it will not be displayed.)
The last argument passes coordinate information. Here, the top-left coordinate is passed.
class mainScene extends Phaser.Scene {
create ()
{
this.cameras.main.setSize(600,600);
this.add.rectangle(0, 0, 800, 600, 0xff0000, 0.5).setOrigin(0);
this.scene.add('second', secondScene, true, { x: 600, y: 0 });//Add
}
}
secondScene setting
The display area is set in secondScene
.
The data
parameter receives the object that was passed as the last argument to this.scene.add
in mainScene
.
The display area size is specified by this.cameras.main.setSize
, and the scene’s display area (top-left point) is specified by this.cameras.main.setPosition
.
While I skipped it here, it would be cleaner to get the width
and height
values from mainScene
as well.
class secondScene extends Phaser.Scene {
create (data)//add argument
{
this.cameras.main.setSize(200,600);//add
this.cameras.main.setPosition(data.x,data.y)//add
this.add.rectangle(0, 0, 200, 600, 0x00ff00, 0.5).setOrigin(0);
}
}
Result
We are able to display mainScene (red) and secondScene (green) side-by-side, as in the design diagram.
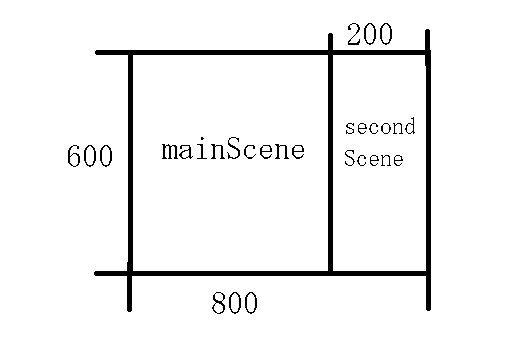
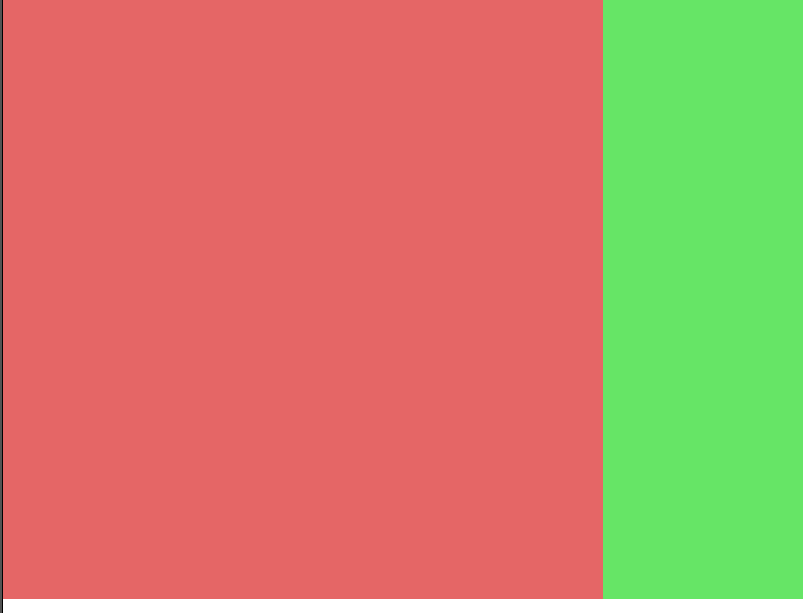
comment