The purpose
In Phaser 3, you can register click events to GameObjects and use them as buttons.
However, with GameObjects, it is difficult to create complex buttons other than using images with simple shapes like rectangles or circles.
Here, we will create sophisticated(Rounded) buttons using graphics objects.
Code
The following function has been created.
function createRoundedRectangle(scene, x, y, width, height, radius, fill, stroke, strokeThickness) {
const graphics = scene.add.graphics();
graphics.fillStyle(fill);
graphics.lineStyle(strokeThickness, stroke);
graphics.beginPath();
graphics.moveTo(x + radius, y);
graphics.lineTo(x + width - radius, y);
graphics.arc(x + width - radius, y + radius, radius, Math.PI * 1.5, 0, false);
graphics.lineTo(x + width, y + height - radius);
graphics.arc(x + width - radius, y + height - radius, radius, 0, Math.PI * 0.5, false);
graphics.lineTo(x + radius, y + height);
graphics.arc(x + radius, y + height - radius, radius, Math.PI * 0.5, Math.PI, false);
graphics.lineTo(x, y + radius);
graphics.arc(x + radius, y + radius, radius, Math.PI, Math.PI * 1.5, false);
graphics.closePath();
graphics.fillPath();
graphics.strokePath();
graphics.setInteractive(new Phaser.Geom.Rectangle(x, y, width, height), Phaser.Geom.Rectangle.Contains);
return graphics;
}
Be careful that graphics.setInteractive
does not work if you call it without any arguments, unlike other objects.
Call
The above function is called from within the Scene class like this:
let x = 100;
let y = 200;
let font_size = 10;
let fill_color = 0xffffff;
let stroke_color = 0x000000;
let strokeThickness = 3;
let func = () =>{alert()};
createRoundedRectangle(this, x, y, width, height, radius, fill_color, stroke_color, strokeThickness).on('pointerup', func);
Result
I was able to create elaborate buttons without using images.
Note that this function does not display the string, so if you want to show the characters, you need to display them separately.
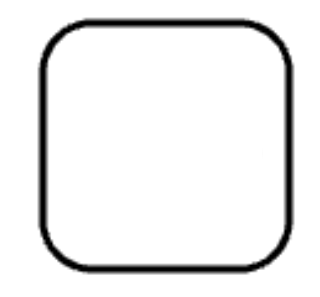
comment