The purpose
read and write values between different Scenes in Phaser 3.
As an example, I’ll use the code that displays two scenes side-by-side, created using the instructions on the page below.
The code is below.
class secondScene extends Phaser.Scene {
create (data)
{
this.cameras.main.setSize(200,600);
this.cameras.main.setPosition(data.x,data.y)
this.add.rectangle(0, 0, 200, 600, 0x00ff00, 0.5).setOrigin(0);
}
}
class mainScene extends Phaser.Scene {
create ()
{
this.cameras.main.setSize(600,600);
this.add.rectangle(0, 0, 800, 600, 0xff0000, 0.5).setOrigin(0);
this.scene.add('second', secondScene, true, { x: 600, y: 0 });
}
}
Executing the program displays mainScene, which shows a red rectangle on the left, and secondScene, which shows a green rectangle on the left.
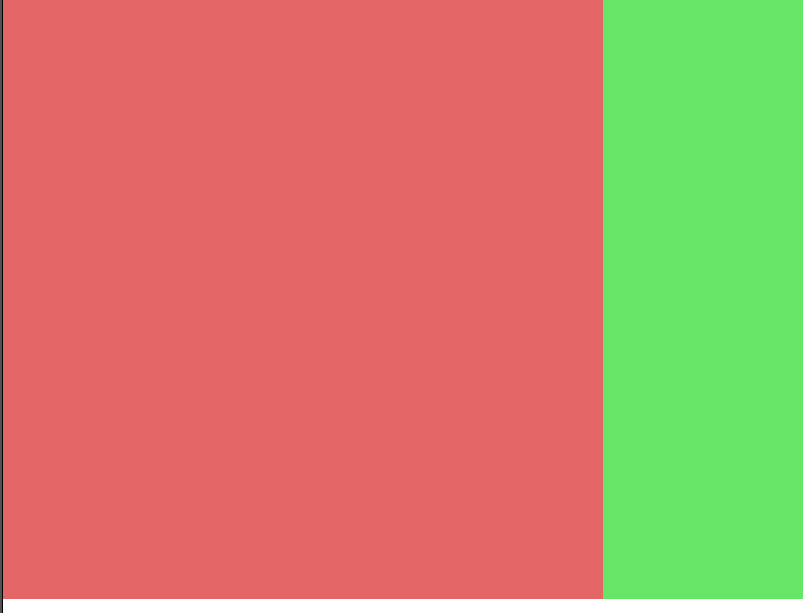
Implementation
Try
implement it according to the following specifications.
- Increment the count in the
secondScene
‘supdate
method. - Retrieve the count from
secondScene
inmainScene
; reset it to 0 if it exceeds 500. - Display the retrieved count in
mainScene
.
secondScene side implementation
There are no special considerations for secondScene
. (However, in this case, we can’t define count
as private because we need to directly manipulate its value from outside the class.)
Define count
within the class, and increment it (count++
) in the update
method as specified above.
count = 0;
update() {
this.count++;
}
mainScene side implementation
Creating a display area for the count
A Text area is created in the upper left using this.add.text
.
The created Text object is saved to count_text
.
count_text;
create ()
{
this.cameras.main.setSize(600,600);
this.add.rectangle(0, 0, 800, 600, 0xff0000, 0.5).setOrigin(0);
this.scene.add('second', secondScene, true, { x: 600, y: 0 });
this.count_text = this.add.text(0, 0, "0").setOrigin(0); //追加
}
Retrieving and displaying the count
Create an update method in mainScene.
We’ll retrieve the ‘second’ scene using this.scene.get('second')
. ‘second’ is the scene key, which is the first argument in this.scene.add
.
You can obtain the count from the acquired scene. (Implementing a Get method is perfectly fine, of course.)
The acquired count is displayed using this.count_text.setText
.
update(){
let count = this.scene.get('second').count;
this.count_text.setText(count);
}
Running the current code now displays a counter in the upper left corner.
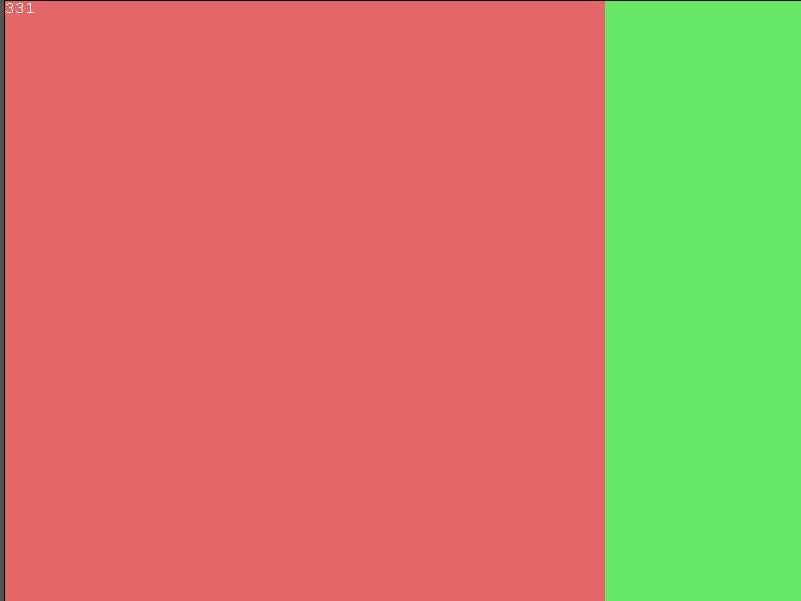
Update count
Count updates can be performed by updating the count
of the scene obtained using this.scene.get
, just like retrieving it. (Implementing a Set
method is also perfectly acceptable.)
To reset the count to 0 when it exceeds 500, as per the specification, I implemented it as follows:
update(){
let count = this.scene.get('second').count;
if(count > 500) { //add start
count = 0;
this.scene.get('second').count =0;
} //add end
this.count_text.setText(count);
}
Result
We are able to read and write values from different scenes.
Another solution
In most cases, other scenes are created elsewhere and need to be retrieved using this.scene.get
.
However, when creating a scene directly with this.scene.add
as in this case, you can save and use the returned value.
class mainScene extends Phaser.Scene {
count_text;
second_scene; //add
create ()
{
this.cameras.main.setSize(600,600);
this.add.rectangle(0, 0, 800, 600, 0xff0000, 0.5).setOrigin(0);
this.second_scene = this.scene.add('second', secondScene, true, { x: 600, y: 0 }); //代入
this.count_text = this.add.text(0, 0, "0").setOrigin(0);
}
update(){
let count = this.second_scene.count; //use value in sub scene
if(count > 500) {
count = 0;
this.second_scene.count = 0; //use value in sub scene
}
this.count_text.setText(count);
}
}
Supplement
The key argument for this.scene.get
is created implicitly, without explicit assignment using methods like this.scene.add
.
class SceneA extends Phaser.Scene {
constructor ()
{
super({ key: 'SceneA'});
}
Or
class SceneA extends Phaser.Scene {
constructor ()
{
super('SceneA');
}
As shown above, the Scene constructor passes arguments to its parent class’s constructor.
comment