The purpose
In the previous article, we set up collision detection for images with complex shapes. Here, however, we’ll use Matter.js to create collision detection for basic shapes (circles, rectangles, and polygons) without using images.
Implementation
Circle
The following implementation draws a black (0x000000) circle with a radius of 10 at coordinates (300, 30).
The second line sets the same radius for the collision detection circle.
const circle = this.add.circle(300, 30, 10 ,0x000000);
this.matter.add.gameObject(circle, { shape: { type: 'circle', radius: 10 } });
Rectangle
The following implementation draws a black (0x000000) rectangle with a width of 50 and a height of 20 at the coordinates (100, 200).
The collision detection is set on the second line; however, since the rectangle and collision detection area are the same size in this case, explicitly setting it is unnecessary.
const rect = this.add.rectangle(100,200,50,20,0x000000)
this.matter.add.gameObject(rect/*, { shape: { type: 'rectangle', width: 50, height: 20} }*/);
Polygon
The following implementation draws a polygon in black (0x000000) at (400, 300).
The polygon’s vertices are specified by the points
array.
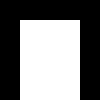
In the last line, I’m setting it to a polygon whose collision detection is created with the same coordinate points.
const points = '0 0 ' +
'100 0 ' +
'100 100 ' +
'80 100 ' +
'80 20 ' +
'20 20 ' +
'20 100 ' +
'0 100 ' +
'0 0';
const poly = this.add.polygon(400, 300, points, 0x000000);
this.matter.add.gameObject(poly, { shape: { type: 'fromVerts', verts: points, flagInternal: true } });
Result
We are able to place shapes with collision detection as follows. (The y-values are changing due to gravity.)
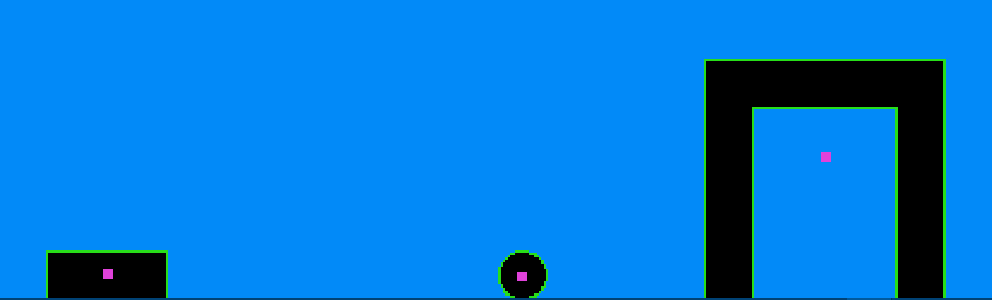
comment