The purpose
run the tutorial game for Phaser 3, a 2D game creation engine.
Start tutorial game
Download code
Download the source code (phaser3-tutorial-src.zip) from the page below.
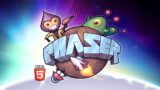
It’s somewhat difficult to see, but there’s a link to download a zip file in the Requirements section.
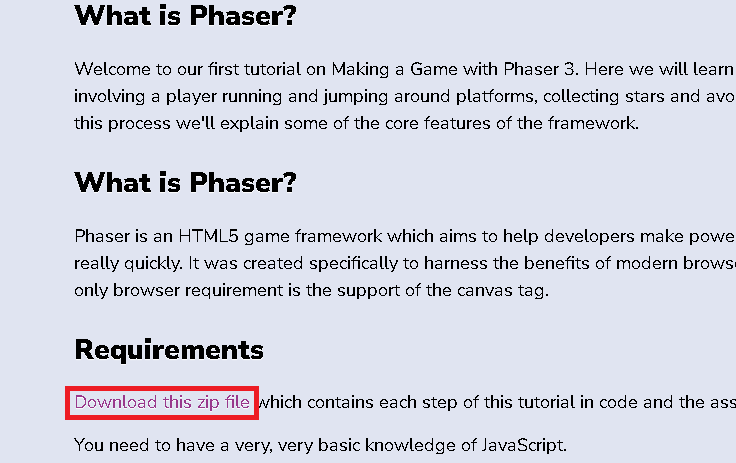
Execute
Extract phaser3-tutorial-src.zip
to a location of your choice.
We will then launch it using Visual Studio Code’s Live Server.
“Double-clicking the HTML file or loading it directly in a browser will not run the game.
(This is because loading external files results in an error.)”
For more details, including installation instructions, please refer to the page below.
Launch Visual Studio Code, select “Open Folder” from the File menu, and open the folder where you extracted the zip file (the folder containing part1.html).
Open the HTML file you want to run, and click “Go live” in the bottom right corner of the Visual Studio Code window.
By selecting Part10.html and clicking “Go live,” I successfully launched the game in Chrome as shown below.
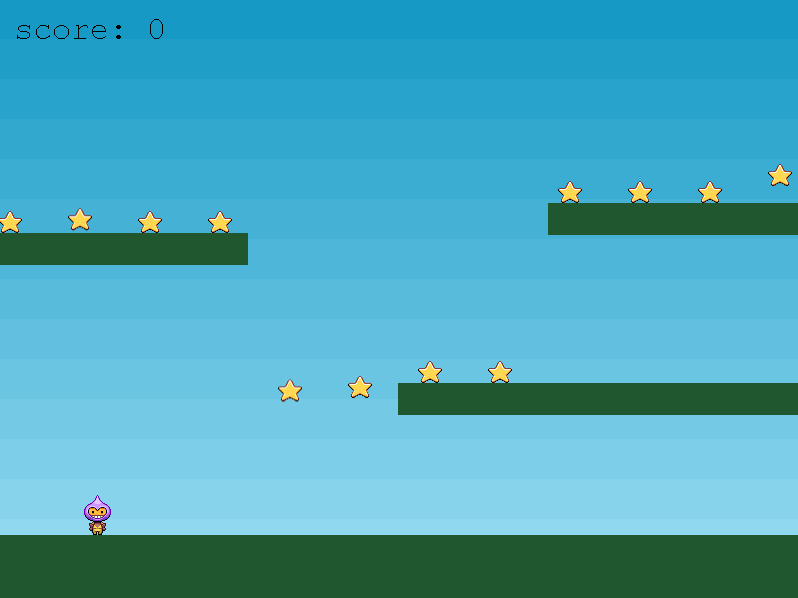
Comments
The target code is the completed Part 10. (With some programming experience, this alone should be enough to create a certain type of game.)
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Making your first Phaser 3 Game - Part 10</title>
<script src="//cdn.jsdelivr.net/npm/phaser@3.11.0/dist/phaser.js"></script><!-- load Phaser.js from cdn -->
<style type="text/css">
body {
margin: 0;
}
</style>
</head>
<body>
<script type="text/javascript">
var config = {
type: Phaser.AUTO,
width: 800, ////Game width
height: 600, ////Game height
physics: {
default: 'arcade', ////kind of physics engine.
arcade: {
gravity: { y: 300 }, ////gravity down direction 300
debug: false
}
},
scene: {
preload: preload, ////function called before scene load
create: create, ////function called when scene load
update: update ////function called while scene
}
};
var player; ////player object
var stars; ////Star object
var bombs; ////bomb object
var platforms; ////ground object
var cursors; ////input event
var score = 0; ////Score
var gameOver = false; ////whether gameover
var scoreText; ////String for score
var game = new Phaser.Game(config); ////create game
function preload () ////function called before load scene
{
this.load.image('sky', 'assets/sky.png');//// load assets/sky.png as name "sky"
this.load.image('ground', 'assets/platform.png');//// load assets/ground.png as name "ground"
this.load.image('star', 'assets/star.png');//// load assets/star.png as name "star"
this.load.image('bomb', 'assets/bomb.png');//// load assets/bomb.png as name "bomb"
this.load.spritesheet('dude', 'assets/dude.png', { frameWidth: 32, frameHeight: 48 });//// load assets/dude.png as name "dude" this is sprite has 32x48 images
}
function create ()
{
// A simple background for our game
this.add.image(400, 300, 'sky');//add sky(400,300) center of game
// The platforms group contains the ground and the 2 ledges we can jump on
platforms = this.physics.add.staticGroup();// create ground group
// Here we create the ground.
// Scale it to fit the width of the game (the original sprite is 400x32 in size)
platforms.create(400, 568, 'ground').setScale(2).refreshBody();//create ground with zoom
// Now let's create some ledges
platforms.create(600, 400, 'ground');//create platform
platforms.create(50, 250, 'ground');//create platform
platforms.create(750, 220, 'ground');//create platform
// The player and its settings
player = this.physics.add.sprite(100, 450, 'dude');//
// Player physics properties. Give the little guy a slight bounce.
player.setBounce(0.2);///
player.setCollideWorldBounds(true);//
// Our player animations, turning, walking left and walking right.
this.anims.create({////animation for move left
key: 'left',
frames: this.anims.generateFrameNumbers('dude', { start: 0, end: 3 }),////Splite”dude”の0-3
frameRate: 10,
repeat: -1
});
this.anims.create({////animation for stop
key: 'turn',
frames: [ { key: 'dude', frame: 4 } ],
frameRate: 20
});
this.anims.create({////animation for move right
key: 'right',
frames: this.anims.generateFrameNumbers('dude', { start: 5, end: 8 }),////Splite”dude”5-8
frameRate: 10,
repeat: -1
});
// Input Events
cursors = this.input.keyboard.createCursorKeys();////create input event
// Some stars to collect, 12 in total, evenly spaced 70 pixels apart along the x axis
stars = this.physics.add.group({//create star group
key: 'star',
repeat: 11, //11
setXY: { x: 12, y: 0, stepX: 70 }///each 70px
});
stars.children.iterate(function (child) {///for each star
// Give each star a slightly different bounce
child.setBounceY(Phaser.Math.FloatBetween(0.4, 0.8));///put stars at random
});
bombs = this.physics.add.group();///create bomb group
// The score
scoreText = this.add.text(16, 16, 'score: 0', { fontSize: '32px', fill: '#000' });///create score display
// Collide the player and the stars with the platforms
this.physics.add.collider(player, platforms);///create collider between player and platforms
this.physics.add.collider(stars, platforms);///create collider between stars and platforms
this.physics.add.collider(bombs, platforms);///create collider between bombs and platforms
// Checks to see if the player overlaps with any of the stars, if he does call the collectStar function
this.physics.add.overlap(player, stars, collectStar, null, this);///for get star
this.physics.add.collider(player, bombs, hitBomb, null, this);///for gameover
}
function update ()//this function is called while scene sequentially
{
if (gameOver)//after gameover
{
return;
}
if (cursors.left.isDown)// left key is pressing
{
player.setVelocityX(-160); //move to left
player.anims.play('left', true);//play animation
}
else if (cursors.right.isDown)//right key is pressing
{
player.setVelocityX(160); //move to right
player.anims.play('right', true);//play animation
}
else
{
player.setVelocityX(0);
player.anims.play('turn');
}
if (cursors.up.isDown && player.body.touching.down)//upkey is pressing/player is not in air
{
player.setVelocityY(-330); //jump
}
}
function collectStar (player, star)//overlap star and plaayer
{
star.disableBody(true, true);//remove star
// Add and update the score
score += 10;//update score
scoreText.setText('Score: ' + score);
if (stars.countActive(true) === 0)///
{
// A new batch of stars to collect
stars.children.iterate(function (child) {
child.enableBody(true, child.x, 0, true, true);//add star
});
var x = (player.x < 400) ? Phaser.Math.Between(400, 800) : Phaser.Math.Between(0, 400);//
var bomb = bombs.create(x, 16, 'bomb');//Create bomb
bomb.setBounce(1);//
bomb.setCollideWorldBounds(true);//
bomb.setVelocity(Phaser.Math.Between(-200, 200), 20);//
bomb.allowGravity = false;//ignore gravity
}
}
function hitBomb (player, bomb)//for game over
{
this.physics.pause();//
player.setTint(0xff0000);//make player red
player.anims.play('turn');//stop animation
gameOver = true;//turn on gameover flag
}
</script>
</body>
</html>
comment