The purpose
simple way to load glTF (.glb) files into Three.js; these files are recommended by both Three.js and Microsoft.
environment
Threejs: r150
Chrome: 111.0.5563.65
Prepare
Make the module loadable
if you uses local server for debug, this step is not needed.
This uses JavaScript modules with the type="module"
attribute. For local use, you need to launch Chrome with special flags. A regular Chrome instance cannot load JavaScript modules with type="module"
.
To launch Chrome, add the following arguments:
--allow-file-access-from-files
Several options exist to append the arguments: you can create a Chrome shortcut with the arguments appended to the link, or create a batch file (.bat).
When launching Chrome using the above method, all Chrome windows must be closed.
Create glTF file
load by GLTFLoader
folder structure
The folder structure is as follows:
dice.glb
is the target file to be loaded.
GLTFLoader.js
, three.module.js
, and BufferGeometryUtils.js
are files included in Three.js.
ROOT
| dice.glb
| index.html
|
\---js
+---libs
| 3d.js
| GLTFLoader.js //original (three.js\examples\jsm\loaders¥GLTFLoader.js)
| three.module.js //original (three.js\build\three.module.js)
|
\---utils
BufferGeometryUtils.js //original (three.js\examples\jsm\utils¥BufferGeometryUtils.js)
HTML
contents of index.html is below.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
</head>
<body>
<canvas id="canvas1" width=600 height=600></canvas>
<script type="importmap">{"imports": {"three": "./js/libs/three.module.js"}}</script>
<script type="module" src="./js/libs/3d.js"></script>
</body>
</html>
Below is the explanation for each line.
<canvas id="canvas1" width=600 height=600></canvas>
A Canvas for displaying 3D graphics. An ID is set for JavaScript use.
<script type="importmap">{"imports": {"three": "./js/libs/three.module.js"}}</script>
This is a direct translation of your request, suitable for a technical document.
I struggled a lot without setting this up.
<script type="module" src="./js/libs/3d.js"></script>
Loads JavaScript including a load processing function.
It seems the latest version of three.js only includes a module version of the GLTFLoader for loading glb files. Other websites may provide information about non-module versions, which can lead to incorrect functionality.
JavaScript
import * as THREE from 'three';
import { GLTFLoader } from './GLTFLoader.js';
let renderer;
let scene;
let camera;
scene = new THREE.Scene();
const canvas = document.querySelector("#canvas1");
renderer = new THREE.WebGLRenderer({ canvas: canvas});
const width = canvas.width;
const height = canvas.height;
renderer.setSize(width, height);
setupCamera();
loadObjects(scene,"./dice.glb");
setupLights(scene);
function setupCamera() {
const canvas = document.querySelector("#canvas1");
const width = canvas.width;
const height = canvas.height;
camera = new THREE.PerspectiveCamera(45, width / height, 0.1, 3000);
camera.position.set(4, 4, 4);
camera.lookAt(new THREE.Vector3(0, 0, 0));
}
function render() {
renderer.render(scene, camera);
}
function setupLights(scene) {
let ambientLight = new THREE.AmbientLight(0xffffff);
ambientLight.intensity = 1;
scene.add(ambientLight);
}
function loadObjects(scene, path) {
const loader = new GLTFLoader();
loader.load(path, function(gltf) {
let box = gltf.scene;
scene.add(box);
requestAnimationFrame(render);
}, undefined, function(e) {
console.error(e);
});
}
The process for loading a .glb file is as follows. Other processes are typical Three.js image display processes.
import { GLTFLoader } from './GLTFLoader.js';
Import the loader for loading .glb
files.
function loadObjects(scene, path) {
const loader = new GLTFLoader();
loader.load(path, function(gltf) {
let box = gltf.scene;
scene.add(box);
requestAnimationFrame(render);
}, undefined, function(e) {
console.error(e);
});
}
Set the path to the .glb
file to be loaded in the path
variable. If using a relative path, specify it relative to the HTML file.
The function in the second argument of loader.load
is called when loading is complete. Here, gltf.scene
from the loading result is added to the scene
using add()
. After adding, requestAnimationFrame()
is used to request rendering and display the scene on the screen.
gltf.scene is a group, not a Three.js scene. It cannot be rendered directly.
This is a concise and accurate translation.
Result
We are able to load and display dice.glb.
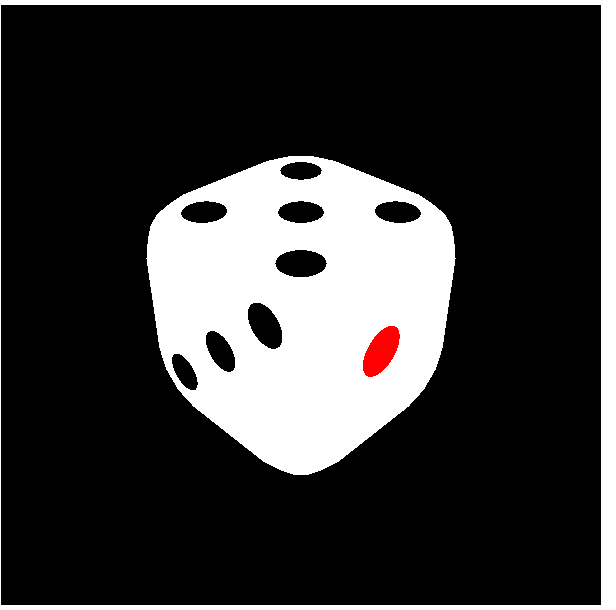
Reference
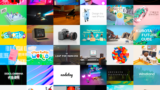
comment