The purpose
This reads a locally stored text file using JavaScript.
See below for information on drag-and-drop file loading.
Reading using the Filesystem API
By clicking the “load text” button in the HTML below, you can select and load a file from the file selector. The loaded data will be stored in text
.
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8" />
<title>load text</title>
</head>
<body>
<input type=button id="load" value="load text">
<script>
async function loadText() {
const opt = {
types: [
{
accept: { "text/plain": [".txt"] }
}
]
};
const handle = await window.showOpenFilePicker(opt);
if (handle[0] != undefined) {
const file = await handle[0].getFile();
const text = await file.text();
console.log(text);
}
}
document.getElementById("load").onclick = loadText;
</script>
</body>
</html>
The following Opt
are read options. In this example, we’re targeting text files, so we’ve set "text/plain": [".txt"]
. For a list of common MIME types, please refer to the following page.
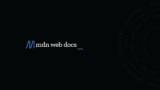
const opt = {
types: [
{
accept: { "text/plain": [".txt"] }
}
]
};
The following line displays the file selector.
const handle = await window.showOpenFilePicker(opt);
showOpenFilePicker
must be called immediately after a user operation, such as a click. For example, performing heavy processing or pausing for debugging between a click and calling showOpenFilePicker
will result in an error like the one described below and cause the function to fail. (Directly calling showOpenFilePicker
or loadText
without a preceding user interaction is also not allowed.)
Uncaught (in promise) DOMException: Failed to execute ‘showOpenFilePicker’ on ‘Window’: Must be handling a user gesture to show a file picker.
at HTMLInputElement.loadText
The following code reads the contents of a file. The contents are stored as a String in the text
variable.
In this example, only one file can be selected, but the handle is an array.
In this example, the file content is fixed as Text, so we are reading it using file.text()
. For binary data, we use file.arrayBuffer()
.
const handle = await window.showOpenFilePicker(opt);
if (handle[0] != undefined) {
const file = await handle[0].getFile();
const text = await file.text();
console.log(text);
}
Result
We are able to read a locally saved text file using a file selector.
comment