The purpose
Get the SHA-256 hash value in JavaScript.
Implementation
A hash can be retrieved using the following function.
async function digestMessage(message) {
const msgUint8 = new TextEncoder().encode(message); // encode message to Uint8Array
const hashBuffer = await crypto.subtle.digest("SHA-256", msgUint8); //create hash
const hashArray = Array.from(new Uint8Array(hashBuffer)); // buffer to byte array
const hashHex = hashArray.map((b) => b.toString(16).padStart(2, "0")).join(""); // byte array to hex string
return hashHex;
}
The caller should do the following. (Since it’s an async function, you need to use await
or .then()
to receive the return value.)
const str ="ハッシュを求める文字列";
const hash = await digestMessage(str);
Besides “SHA-256”, “SHA-1”, “SHA-384”, and “SHA-512” can be specified for crypto.subtle.digest
. However, “SHA-1” is prohibited for cryptographic use.
Result
We got a hash value using JavaScript.
'e5f8d3681f38da0737857b4b0136362fdd62f3558274a54cb07c32f63143d203'
Reference
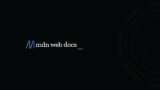
SubtleCrypto: digest() メソッド - Web API | MDN
digest() は SubtleCrypto インターフェイスのメソッドで、指定されたデータのダイジェストを返します。ダイジェストとは、可変長の入力に由来する固定長の短い値です。暗号的ダイジェスト値は耐衝突性を示すため、同じダイジェスト値を持つ 2 つの異なる入力を見つけるのは非常に困難です。
comment