The purpose
This site previously introduced Three.js based on modules; however, we’ll now examine the differences between non-modular and modular scripts.
The Three.js Example/js folder has been removed (unless I’m mistaken), making it impossible to use Loaders and Extensions unless they are modular. Modularization will likely become essential going forward.
Environment
Threejs: r150
Ready to run in the module
Make the module importable
*if you use local server like a node.js, this step is not needed.
Type modules use JavaScript modules. If using locally, you need to launch Chrome with a special flag. Regular Chrome can’t load Type module JavaScript.
Add the following arguments when launching Chrome:
--allow-file-access-from-files
It’s convenient to create a Chrome shortcut and append the above arguments to the end of the link, or to create a .bat file.
When launching Chrome using the method described above, all Chrome windows must be closed.
code without module
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
</head>
<body>
<canvas id="canvas1" width=600 height=600></canvas>
<script src ="./js/libs/three.min.js"></script>
<script src ="./js/libs/3d.js"></script>
</body>
</html>
JavaScript
3d.js
let renderer;
let scene;
let camera;
scene = new THREE.Scene();
const canvas = document.querySelector("#canvas1");
renderer = new THREE.WebGLRenderer({ canvas: canvas });
const width = canvas.width;
const height = canvas.height;
renderer.setSize(width, height);
setupCamera();
setupObject(scene);
setupLights(scene);
function setupCamera() {
const canvas = document.querySelector("#canvas1");
const width = canvas.width;
const height = canvas.height;
camera = new THREE.PerspectiveCamera(45, width / height, 0.1, 3000);
camera.position.set(0, 10, 0);
camera.lookAt(new THREE.Vector3(0, 0, 0));
}
function render() {
renderer.render(scene, camera);
}
function setupLights(scene) {
const light = new THREE.DirectionalLight(0xFFFFFF);
light.intensity = 1;
light.position.set(10, 20, -20);
scene.add(light);
}
function setupObject(scene) {
const geometry = new THREE.SphereGeometry(2, 32, 32);
const material = new THREE.MeshStandardMaterial({ color: 0xffff00 });
const sphere = new THREE.Mesh(geometry, material);
scene.add(sphere);
requestAnimationFrame(render);
}
code with module
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
</head>
<body>
<canvas id="canvas1" width=600 height=600></canvas>
<script type="importmap">{"imports": {"three": "./js/libs/three.module.js"}}</script>
<script type="module" src="./js/libs/3d.js"></script>
</body>
</html>
JavaScript
3d.js
import * as THREE from 'three';
let renderer;
let scene;
let camera;
scene = new THREE.Scene();
const canvas = document.querySelector("#canvas1");
renderer = new THREE.WebGLRenderer({ canvas: canvas });
const width = canvas.width;
const height = canvas.height;
renderer.setSize(width, height);
setupCamera();
setupObject(scene);
setupLights(scene);
function setupCamera() {
const canvas = document.querySelector("#canvas1");
const width = canvas.width;
const height = canvas.height;
camera = new THREE.PerspectiveCamera(45, width / height, 0.1, 3000);
camera.position.set(0, 10, 0);
camera.lookAt(new THREE.Vector3(0, 0, 0));
}
function render() {
renderer.render(scene, camera);
}
function setupLights(scene) {
const light = new THREE.DirectionalLight(0xFFFFFF);
light.intensity = 1;
light.position.set(10, 20, -20);
scene.add(light);
}
function setupObject(scene) {
const geometry = new THREE.SphereGeometry(2, 32, 32);
const material = new THREE.MeshStandardMaterial({ color: 0xffff00 });
const sphere = new THREE.Mesh(geometry, material);
scene.add(sphere);
requestAnimationFrame(render);
}
Diffence
HTML
Without module
<script src ="./js/libs/three.min.js"></script>
<script src ="./js/libs/3d.js"></script>
With module
<script type="importmap">{"imports": {"three": "./js/libs/three.module.js"}}</script>
<script type="module" src="./js/libs/3d.js"></script>
For projects without module, include three.min.js
(or three.js
) in your HTML.
For projects with module, you need to specify an import map (as mentioned in the first point).
The path "./js/libs/three.module.js"
needs to be adjusted based on your environment. Set the “module” type for your Javascript file.
JavaScript
The module requires the following line
import * as THREE from 'three';
There are no other differences.
In this example, the Three.js class is loaded using the THREE namespace, so no modifications other than the import statement are necessary. However, the way the import is written may require a namespace change.
Result
In the example above, yellow spheres are displayed in both the modular and non-modular cases.
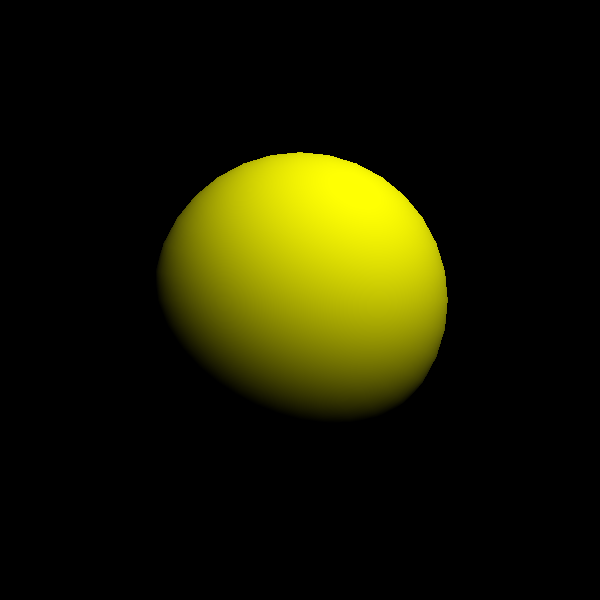
comment