The purpose
The following article uses buttons set via the Inspector.
However, since the buttons are not used within the class after the callback is set, there’s no need to keep them as members. Furthermore, experienced programmers will find setting buttons through the GUI cumbersome (it’s also difficult to identify which button is being set just by looking at the code).
Therefore, this article introduces a method to obtain Button objects directly from the code.
Previous code
In the preceding article, I wrote the following code.
m_Button
is set via the Inspector.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Example : MonoBehaviour
{
public Button m_Button;
void Start()
{
m_Button.onClick.AddListener(() => ButtonClicked(42));//register function for click. 42 is test value
}
void ButtonClicked(int buttonNo)//// argument can has any type
{
Debug.Log("Button clicked = " + buttonNo);///Output log
/*process for click*/
}
}
Implementation
The following changes will be made:
The member variable m_Button
will be removed.
A GameObject will be obtained using GameObject.Find("ButtonName")
.
“ButtonName” is the name set in the Inspector.
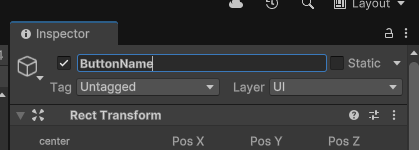
A button component is retrieved from a GameObject.
This component can call ok_btn.onClick.AddListener
, similar to a Button set externally.
public class Example : MonoBehaviour
{
void Start()
{
Button ok_btn = GameObject.Find("ButtonName").GetComponent<Button>();
ok_btn.onClick.AddListener(() => ButtonClicked(42));
}
void ButtonClicked(int buttonNo)////argument can has any type
{
Debug.Log("Button clicked = " + buttonNo);///Output log
/*process for click*/
}
}
Aside
You can obtain other instances besides Buttons.
For example, with a RawImage, you would do the following. RawImage_Name
is set in the Inspector, just like the Button’s name.
GameObject.Find("RawImage‗Name").GetComponent<RawImage>();
Result
I was able to obtain a Button and set a callback for it using only script, without setting it up in the Inspector.
comment