The purpose
I will create an HTTP server using Node.js.
The implementation will use ESM (import) instead of CJS (require).
Environment
install Node.js
download and install Node.js from the link below, selecting the version appropriate for my environment.
Node.js — Download Node.js®
Node.js® is a JavaScript runtime built on Chrome's V8 JavaScript engine.
Implementation
Create an arbitrary folder and create a file named server.mjs
inside it with the following contents:
import http from 'node:http';
/////Start server, return json for a request
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({
data: 'Hello World!',
}));
});
server.listen(8000);///// use Port8000
Ececute
Open the command prompt and navigate to the folder where you created server.mjs
.
Then, execute the following command.
node .\server.mjs
Operational check
Accessing 127.0.0.1:8000
in a browser like Chrome returns JSON data.
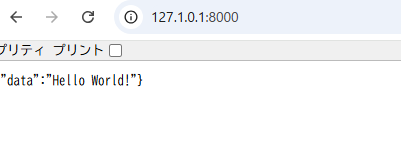
The following returns the same result even if arbitrary characters are entered after 127.0.0.1:8000/
; no 404 or other errors are returned.
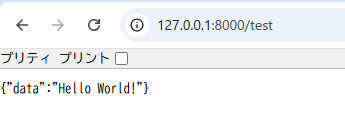
Result
now running an HTTP server with Node.js.
Reference
HTTP | Node.js v23.1.0 Documentation
comment