The purpose
When you want to dynamically import a module, you use an import
function instead of an import
statement.
However, simply writing it didn’t work as expected. Let’s fix it to work correctly.
Review
Before
The following import statements will be rewritten as import functions.
import { myClass } from './myClass.js';
The myClass
class is imported from myClass.js
.
Bad Example
The following code didn’t work as expected.
const myClass= import ('./myClass.js')
Code works well
In conclusion, doing the following resulted in myClass having the same value as before the correction.
const myClass= (await import('./myClass.js'))['myClass'];
Explanation
Here’s an explanation of why the bad example didn’t work.
It stems from a double misunderstanding of the return value of import()
.
The first misunderstanding is that import()
is an async function and its return value is a Promise.
Therefore, I added await
to synchronize it. (Of course, receiving the return value with then
is also fine.)
Considering the above, I made the following correction, but it still doesn’t work.
const myClass= await import('./myClass.js');
Here’s the second misunderstanding.
The promise
above returns an object containing all exports, not just the desired class. (All exports are bundled together, regardless of whether there’s only one.)
Therefore, you need to specify the required class name using ['myClass']
as shown below.
const myClass= (await import('./myClass.js'))['myClass'];
Result
We are able to replace the import
statement with an import
function.
Reference
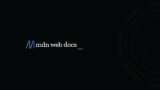
comment