The purpose
The <canvas>
tag’s size can be specified in two ways; while visually identical, their behavior differs when handled by JavaScript, so let’s verify this.
Environment
Chrome: 113.0.5672.127
How to set the canvas size
Canvas size can be set using attributes and styles;
the visual result is the same in both cases.
Settings within the canvas tag’s attributes
<canvas width=200 height=200></canvas>
The HTML above allows a 200×200 Canvas to be drawn as shown below. (The background is grayed out for better visualization of the area.)
Settings in Style
<canvas style="width:200px; height:200px;"></canvas>
With the above HTML, a 200×200 Canvas can be drawn as shown below.
(The background is grayed for better visibility of the area.)
Operated using JavaScript
Coordinate
Test
Let’s try running the following script on a canvas whose size is set using both the attribute
and style
properties.
We’ll draw a blue line from (0,0) to (100,100).
Since the canvas size is 200×200, the blue line should be drawn from the top-left corner to the center.
/*get Context(let ctx) from canvas */
ctx.beginPath() ;
ctx.moveTo( 0, 0 ) ;
ctx.lineTo( 100, 100 )
ctx.strokeStyle = "blue" ;
ctx.lineWidth = 5 ;
ctx.stroke() ;
attribute
style
Result
When the attribute is set as shown above, the expected behavior is observed. However, when specified via the style attribute, the expected behavior is not observed.
The meaning of each Width/Height
Here’s an explanation of why the two Width/Height setting methods produced different results.
In short, the Width/Height values set via attributes and via Style differ.
The meanings of Width/Height in attribute and Style are as follows:
attribute : Width/height of the coordinate space
Style : Display width/height
That means setting the Width/Height with the style
attribute doesn’t change the canvas coordinate system.
If you don’t set the Width/Height with an attribute
, the coordinate space defaults to 300×150.
Therefore, in the following example, a 300×150 canvas is transformed and displayed as 200×200.
<canvas style="width:200px; height:200px;"></canvas>
Therefore, it didn’t produce the expected results.
Conclusion
When setting the size of coordinates within a canvas, you specify it using attributes.
However, this doesn’t mean setting it via style is unnecessary.
For example, changing the size with attributes requires modifying your JavaScript drawing logic.
In contrast, changing the size with style only involves scaling; the content is simply resized.
An implementation where you specify the canvas coordinate size with attributes and then adjust the display size with style to match the window size is also possible.
Reference
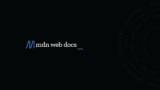
comment