The purpose
create a Windows application using Electron.
about electron
Electron allows you to create executable files from web pages built with JavaScript, HTML, and CSS.
While it can create executables for Windows, macOS, and Linux, this example will focus on Windows.
See the official documentation for more details:
Create proect
Prepare
Node.js is needed.
Install the appropriate Node.js version for your environment from the link below.
Create project
Create a new working folder and run the following command.
npm init -y
Execute on Electron
Install Electron
Install
Open the command prompt and run the following command in the working folder.
npm i electron --save-dev
Preparing the executable file
I’ll create a folder named “Src” under the folder containing the files to be modified into executable files. (For example, I’ll copy the entire contents of the dist
folder bundled by Vite into an arbitrary folder.)
The folder structure will be maintained. The two files prepared this time are located in the same folder.
index.html
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>ELECTRON TEST</title>
</head>
<body>
<h1>ELECTRON TEST</h1>
<img src="./img.png">
</body>
</html>
img.png
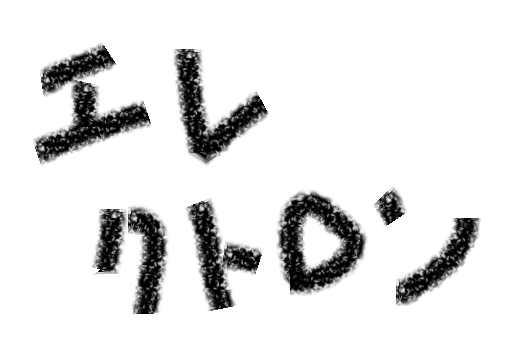
Creating an entry point
Create a new file named main.js
in the same folder where you created the two files mentioned above. (If you need to load files other than index.html
, edit the index.html
part of win.loadFile('file://' + __dirname + '/index.html')
.)
const { app, BrowserWindow } = require('electron/main')
const createWindow = () => {
const win = new BrowserWindow({
width: 800,
height: 600
})
win.loadFile('file://' + __dirname + '/index.html');
}
app.whenReady().then(() => {
createWindow()
app.on('activate', () => {
if (BrowserWindow.getAllWindows().length === 0) {
createWindow()
}
})
})
app.on('window-all-closed', () => {
if (process.platform !== 'darwin') {
app.quit()
}
})
While Electron can run with just "index.html"
as the argument for loadFile
, it will fail after packaging.
Execute
Execute the following command in the parent directory of the Src folder (the working directory).
node_modules/.bin/electron .
The following shows the HTML and image I created.
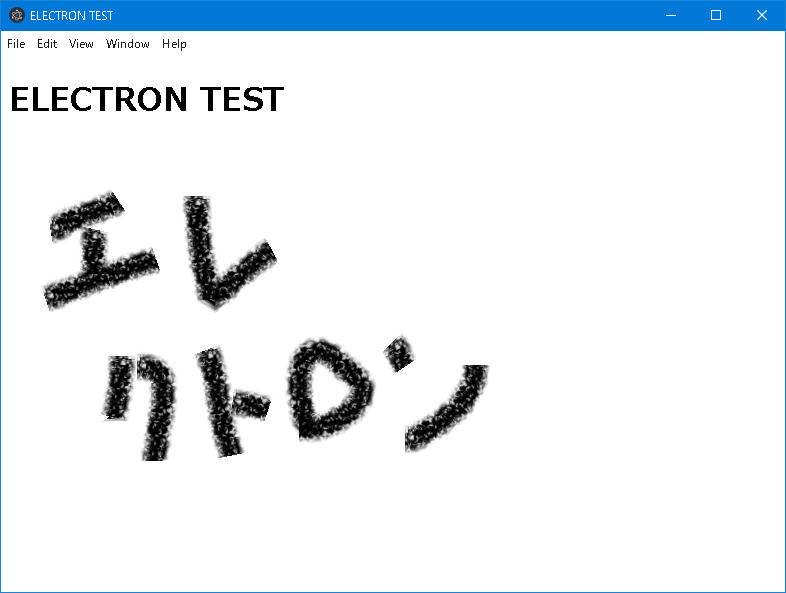
Packaging
Install electron-builder
Install electron-builder in the working directory using the following command.
npm i electron-builder --save-dev
Modify Package.json
Open the Package.json file in the project folder.
modify following file
"main": "index.js",
modify it as follows
"main": "src/main.js",
execute electron-builder
You can build using the following command.
node_modules\.bin\electron-builder build
The process is complete when an executable file is created in the dist
folder. (The exe in dist
is the installer.)
If you encounter the following error, you may not have administrator privileges.
Please run the application used to execute the build command (e.g., Command Prompt, Visual Studio Code) with administrator rights to execute the command.
ERROR: Cannot create symbolic link : �N���C�A���g�͗v�����ꂽ�������ۗL���Ă��܂����B
Result
We are able to create an executable (.exe) file of the web application for Windows.
For your reference
The generated file sizes are as follows. Without optimization, they are near the minimum achievable.
Given the use of Chromium, the size is relatively large.
file size(MByte) | |
installer | 78 |
after install | 264 |
参考にさせていただいたサイト
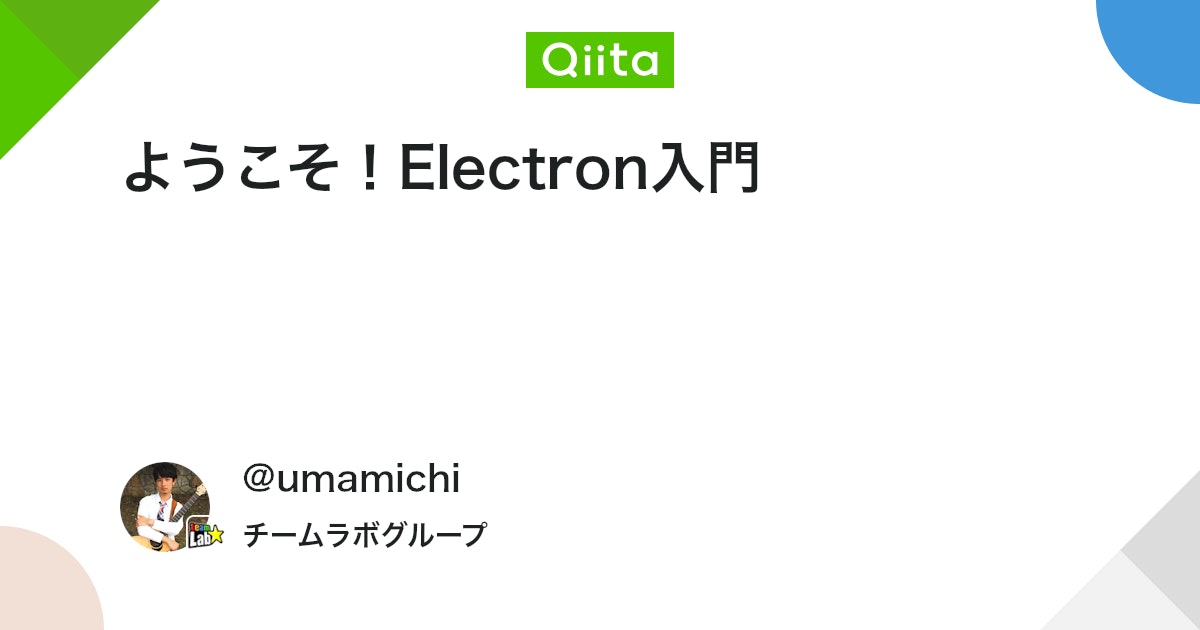
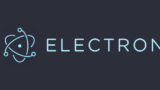
comment