The purpose
Here’s a brief explanation of npm, which you’ll often encounter when researching Node.js and JavaScript/TypeScript.
This summary covers the fundamentals of package management.
about NPM
npm is a package manager for Node.js,
though it offers many functions beyond just package management, such as package execution.
Official page:
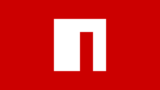
Install
Packages are installed using npm with the following command.
npm install パッケージ名
“Install” can be substituted with any of the following: add, i, in, ins, inst, insta, instal, isnt, isnta, isntal, isntall.
From what I can see, it seems to be either ‘install’ or ‘i’.
Options for Installation Location
local install
Omitting installation options like --save-prod
and -P
will install the package globally. Setting --save-prod
and -P
installs the package into the node_modules
folder within your project.
The installation information is saved in the "dependencies"
section of your package.json
file.
example:
npm install app
local install(dev)
Using --save-dev
and -D
installs the package within the node_modules
folder in your project.
The installation information is saved in the "devDependencies"
section of your package.json
file.
example:
npm install app -D
global install
Using --global
or -G
installs the package outside the project. The path is automatically set, allowing you to run the installed package from anywhere.
example:
npm install app -G
The difference between --save-prod (or no option, which is equivalent) and --save-dev
If you install a package with --save-prod
(or without any save option), it will be included in the final production build. However, if you install it with --save-dev
, it will not be included.
Therefore, libraries referenced from your HTML and JS (like jQuery or three.js) should be installed with --save-prod
(or without a save option), while packages used for development or build processes (such as Electron or TypeScript) should be installed with --save-dev
.
install without argument
If install
is executed without arguments, it will automatically read and install the necessary packages from package.json
and similar files. This is useful for acquiring necessary packages after cloning a template from Git, for example.
npm install
List of installed packages
The following command lists the packages installed via npm.
npm ls
Install can be substituted by any of the items in the list.
Options for installation location
If no arguments are specified, it displays a list of locally installed packages, similar to the install command. (However, it does not distinguish between Dependencies and devDependencies.)
example:
npm ls
Use the -g
option to list globally installed packages.
example:
npm ls -g
uninstall
Packages are installed using the following npm command.
npm uninstall packagename
uninstall
can be replaced with unlink
, remove
, rm
, r
, or un
.
Installed packages can be checked using npm ls
, as explained in “the list of installed packages” (version numbers are displayed but are not necessary).
Packages installed with --save-dev
(-D
) or --global
(-G
) require the same flags (--save-dev
or --global
) when uninstalling.
comment