The purpose
I’m creating FPS-like movement controlled by the mouse in Unity.
I’ll list the creation process and important points to note.
Also, since this is a work in progress, please treat this information as a reference only.
Specification:
Moving the mouse left and right → move view point
left click → Forward
Stop when hit a wall.
Environment
Unity:6000.0.23f1.7976.6000.0/Staging4246
Implementation
Prepare a Player and a camera
Create Player
Create a 3D object (a Cube, for now, since it’s invisible in the FPS view and it’ll suffice; a Cube is also a safe choice for future collision detection) as a child of the Hierarchy’s +.
Move Camera
Drag and drop the MainCamera from the Hierarchy view onto the Player to make it a child of the Player.
The hierarchy should look like this:
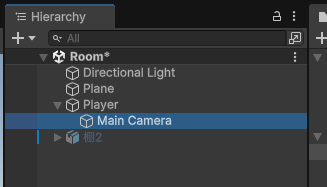
Making the MainCamera a child of the Player automatically makes the camera follow the Player when the Player moves.
As the camera follows the Player, it’s positioned at the same X and Z coordinates as the Player, but slightly above the Player in the Y coordinate.
The cube below represents the Player, and the arrow indicates the camera.
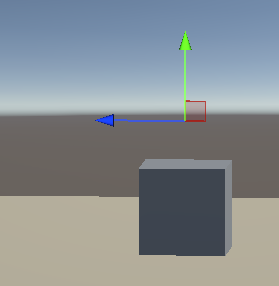
Add script to Player
The Player’s script is as follows:
using UnityEngine;
public class player : MonoBehaviour
{
private float speed = 5.0f;
void Update()
{
float mx = Input.GetAxis("Mouse X");////Get mouse move amonunt
if (Mathf.Abs(mx) > 0.001f)
{
transform.RotateAround(transform.position, Vector3.up, mx); ////update viewpoint
}
if (Input.GetMouseButton(0))//////////repeat process while press left click
{
float zMovement = speed * Time.deltaTime;
transform.Translate(0, 0, zMovement);/////forward
}
}
}
Add Physics
Player setting
In the Player’s Add Component menu, add a Rigidbody component and modify its settings as follows:
Checking “Freeze Position Y” will prevent the object from bouncing upon collision. (This is not mandatory.)
Checking “Freeze Rotation X, Y, and Z” will prevent the object from rotating upon collision. It’s recommended to check these boxes because otherwise the camera will rotate along with the object on collision.
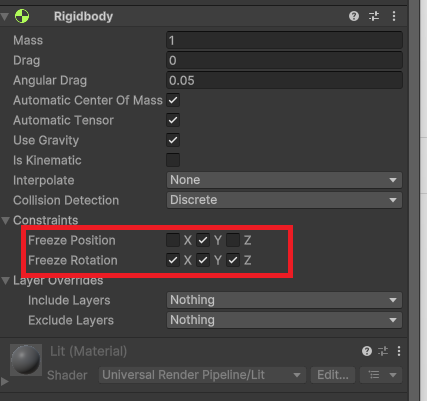
walls/obstacles setting
Use the Blender-created objects from the article below as walls. However, to simplify the physics calculations, the “Generate Collider” option is unchecked.
Select the added object in the Hierarchy view and add a Box Collider.
Then, click Edit Collider.
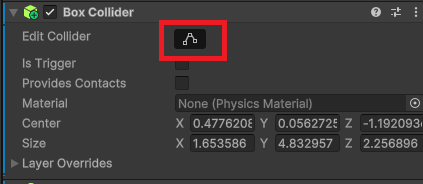
A green bounding box will appear in the Scene view;
align it with your object. (Note that this is different from the blue bounding box that appears when you select an object.)
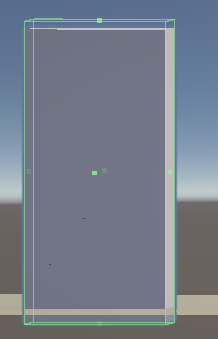
Next, add a Rigidbody component and check all boxes under “Freeze Position” and “Freeze Rotation” as shown below.
This will prevent the wall from moving even upon collision.
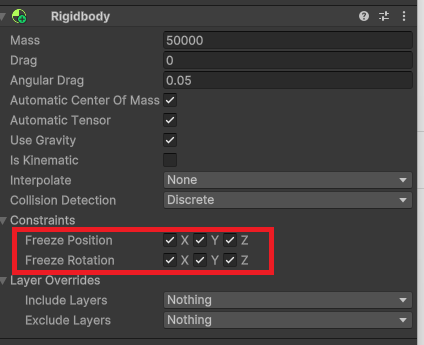
Reference
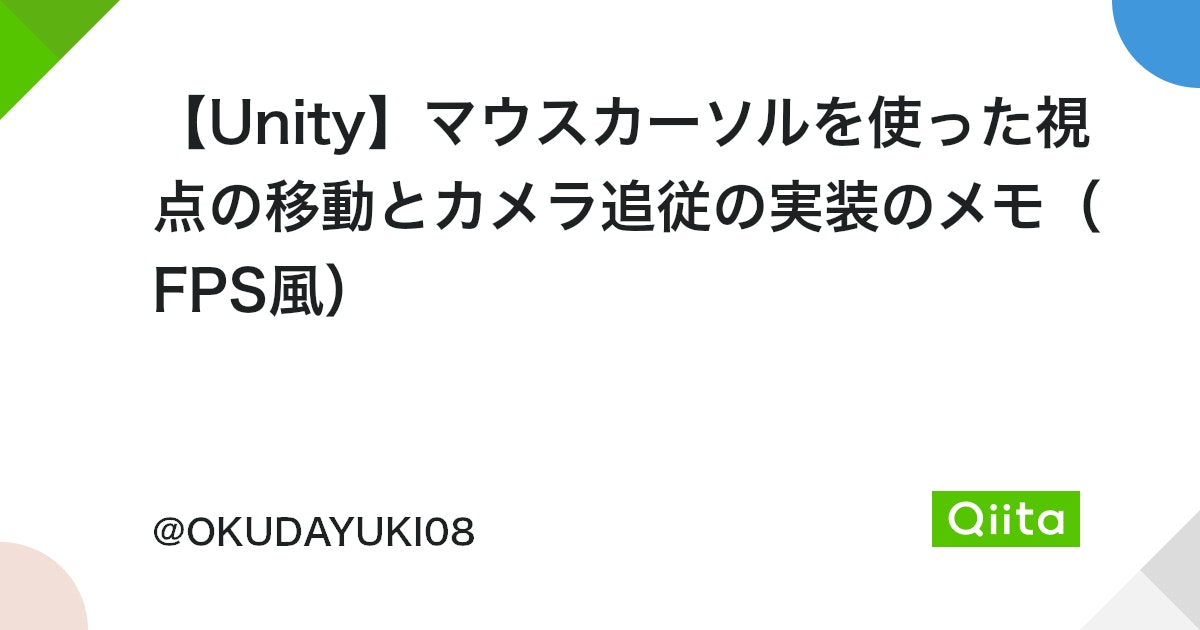
comment