The purpose
Developing with TypeScript (TS) using Visual Studio Code.
(Creating a free GUI-based development environment for TS.)
Environment
OS : Windows11
Visual Studio Code:1.88.1
Node.js : 20.12.2
Build Environment
First, install the necessary tools to set up the development environment.
Install Visual Studio Code
Download and install Visual Studio Code from the link below. (For most PCs, the x64 User Install should be fine.)
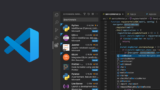
Install Node.js
Download and install Node.js from the link below.
(Node.js is required for the TypeScript development environment.)
Install TypeScript
Run the following command in the Command Prompt.
npm install -g typescript
To verify correct installation, run the following command in the command prompt.
tsc --version
If the version number is displayed, the installation was successful.
Create new project
Create setting file
Create a development folder in any location. For this example, I created the following folder(s).
F:\TS\TEST
Open the command prompt and navigate to the project created above.
F:
cd \TS\TEST
execute the following commands.
npm init -y
npx tsc --init
I created a batch file and attempted to execute it from the File Explorer, but it was unsuccessful.
Success is indicated by the presence of the following two files.
package.json
tsconfig.json
The project template is now complete.
Open project
Open the above settings in Visual Studio Code.
In Visual Studio Code, select “File” -> “Open Folder…” from the menu bar.
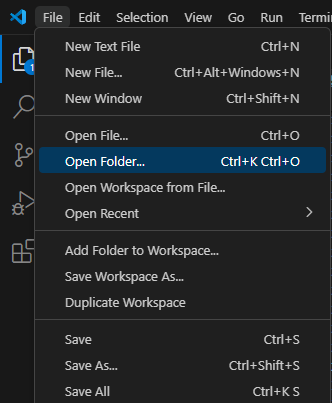
With the folder where you created the settings file (F:\TS\TEST in this case) open, click “Select Folder”. This should open the project.
Change setting
In Visual Studio Code, you’ll see a file list on the left. Open tsconfig.json
. Enable the following settings, which are currently commented out.
"rootDir": "./"
"sourceMap": true
"outDir": "./"
We will also change the values of ‘rootDir’ and ‘outDir’ as follows:
"rootDir": "./src"
"outDir": "./build"
Create code
create the source code.
Since I changed “rootDir” to “./src” earlier, the source code will be created under “./src”.
Specifically, I will create “F:\TS\TEST\src\test.ts”.
The contents of test.ts are as follows:
const test_str:string = "TEST"; ////Assigning 'TEST' to a string variable in TypeScript
console.log(test_str); ////output test_str on console
Build TypeScript
The build files are ready, so I’ll proceed with the build.
Open a terminal (shell) by selecting “View” -> “Terminal” from the top menu.
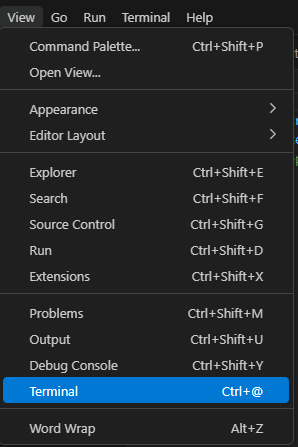
Enter and execute the following command in the displayed terminal.
tsc --build
The build is successful if both “test.js” and “test.js.map” are generated in the “F:\TS\TEST\build” folder.
If you see the following error, your environment may be incorrectly configured.
(the message means “TSC is not recognized as an executable program.”)
tsc : 用語 'tsc' は、コマンドレット、関数、スクリプト ファイル、または操作可能なプログラムの名前として認識されません。名前が正しく記述されていることを確認し、パスが含まれている場合はそのパスが正しいことを確認してから、再試行
してください。
発生場所 行:1 文字:1
+ tsc --version
+ ~~~
+ CategoryInfo : ObjectNotFound: (tsc:String) [], CommandNotFoundException
+ FullyQualifiedErrorId : CommandNotFoundException
Please restart Visual Studio Code. If that doesn’t solve the problem, launch the command prompt and run the following command, then verify that the version is displayed.
If you don’t see a version number, the software may not be installed correctly. Please try reinstalling it.
tsc --version
If the error is displayed, you need to change the settings.
(the message means “cannot read tsc.psm because script is disabled”)
tsc : このシステムではスクリプトの実行が無効になっているため、ファイル C:\Users\xxxxxxx\AppData\Roaming\npm\tsc.ps1 を読み込むことができません。詳細については、「about_Execution_Policies」(https://go.micro
soft.com/fwlink/?LinkID=135170) を参照してください。
発生場所 行:1 文字:1
+ tsc --version
+ ~~~
+ CategoryInfo : セキュリティ エラー: (: ) []、PSSecurityException
+ FullyQualifiedErrorId : UnauthorizedAccess
- Close Visual Studio Code.
- Start Visual Studio Code in administrator mode (right-click the icon and select “Run as administrator”).
- Enter and run the following command in the terminal.
Set-ExecutionPolicy Unrestricted
Once set up, you will no longer need to run it in administrator mode.
I think this setting needs to be changed in Windows 11.
Execute
Open ”test.js”.
Select “Run” → “Start Debugging” from the top menu.
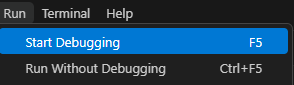
It was confirmed that ‘TEST’ was displayed in the DEBUG CONSOLE.
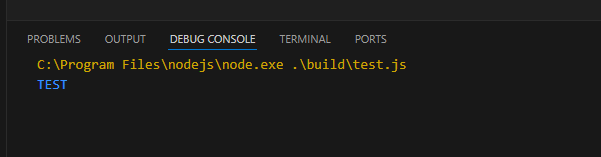
Result
we can now build TypeScript and create/run JavaScript files in Visual Studio Code.
comment