The purpose
Port a Node.js web app into an Android app. (This will not include the server-side/backend.)
use the Node.js + Vite + Phaser 3 template from below.
Implementation
Prepare Web application
Prepare a web app to be made into an Android app.
As shown above, I created a NodeJS + Vite + Phaser 3 environment here and built the web app by running the following command.
npm run build
Following the above procedure, the following files will be created in the dist folder.
dist
| favicon.png
| style.css
| index.html
|
\---assets
bg.png
logo.png
index-C5AljH2-.js
phaser-DJc9ez-r.js
Create Android application project
Open Android Studio and create a new project.
If Android Studio is not installed, please refer to the following link for installation instructions.
For the project, select Empty View Activity, then click Next.
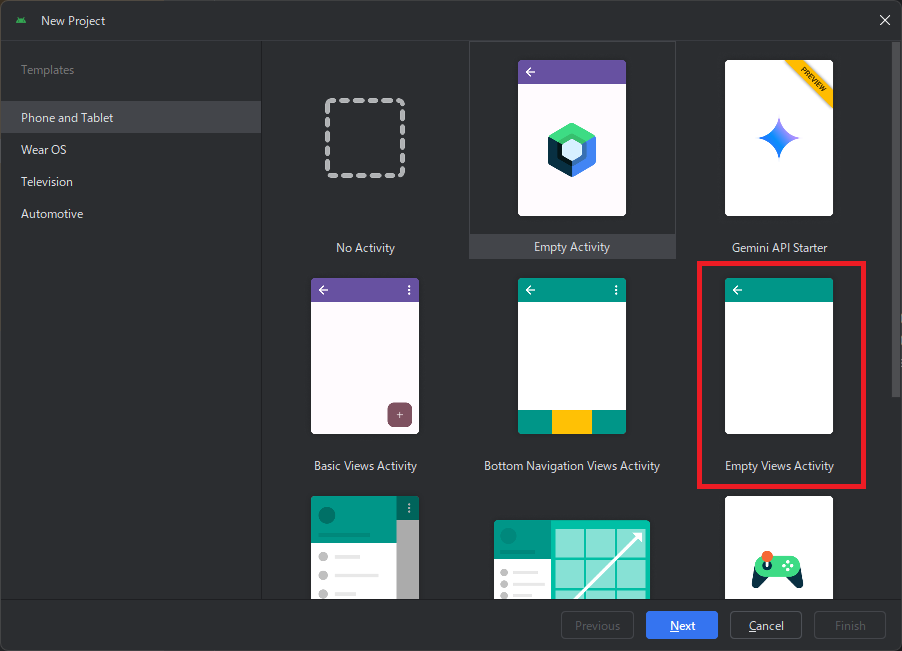
Next, change the Language to Java and click Finish.
Change the other settings to your preferred values according to your environment.
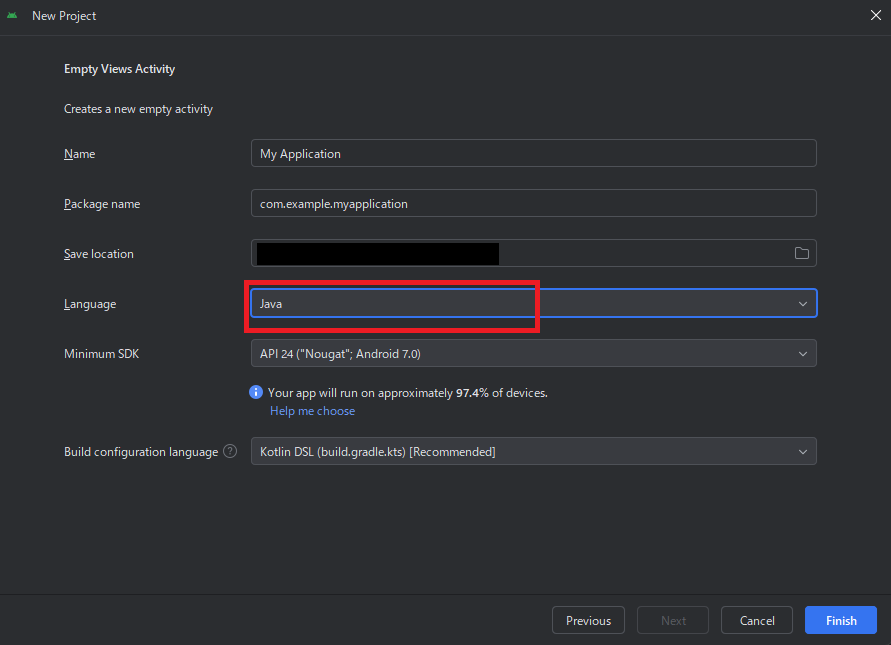
wait while the project is being prepared. (A progress bar will be displayed in the bottom right corner of the window; please wait until it disappears.)
The project preparation is now complete.
Modify layout
Modify the layout to display a WebView.
Open activity_main.xml.
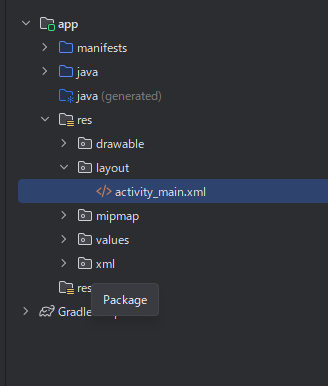
The existing TextView displaying ‘Hello world’ will be removed and a WebView will be placed in its stead.
In my environment, the following results were obtained (with the addition of IDs)
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<WebView
android:id="@+id/webview"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Create Assets folder
Select File → New → Folder → Assets Folder from the menu to create the Assets folder.
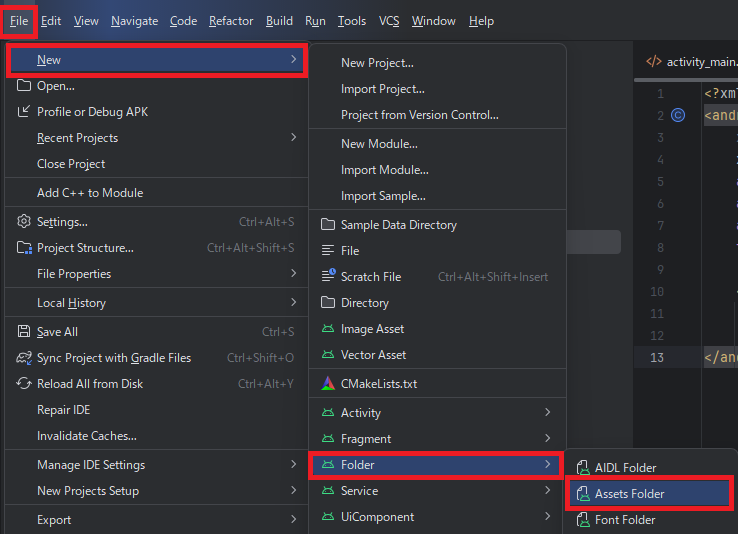
If asked about the target, select Main.
move Web application
An assets
folder will be created in project_root\app\src\main
, into which you should copy the web application you prepared.
The folder structure will then be as follows:
\---assets
| favicon.png
| index.html
| style.css
|
\---assets
bg.png
logo.png
index-C5AljH2-.js
phaser-DJc9ez-r.js
Modify MainActivity
Add the following code to the onCreate()
method of your MainActivity
.
(Import any missing classes as needed. You may need to add the androidx.webkit:webkit
dependency to include WebViewAssetLoader
.)
WebView web_view = ((WebView) findViewById(R.id.webview)); ///レイアウトからwebView取得
WebSettings webSettings = web_view.getSettings(); //WebViewの設定を取得
webSettings.setJavaScriptEnabled(true); //Javascript有効
webSettings.setAllowFileAccessFromFileURLs(false);
webSettings.setAllowUniversalAccessFromFileURLs(false);
webSettings.setAllowFileAccess(false);
webSettings.setAllowContentAccess(false);
final WebViewAssetLoader assetLoader = new WebViewAssetLoader.Builder()
.addPathHandler("/assets/", new WebViewAssetLoader.AssetsPathHandler(this))
.build(); //Create WebViewAssetLoader / Register Assets folder
web_view.setWebViewClient(new WebViewClientCompat() { ///to use assetLoader for web request
@Override
public WebResourceResponse shouldInterceptRequest(WebView view, WebResourceRequest request) {
return assetLoader.shouldInterceptRequest(request.getUrl());
}
@Override
@SuppressWarnings("deprecation") // for API < 21
public WebResourceResponse shouldInterceptRequest(WebView view, String url) {
return assetLoader.shouldInterceptRequest(Uri.parse(url));
}
});
web_view.loadUrl("https://appassets.androidplatform.net/assets/index.html");///load assets/index.html
While it’s conceivable to load the HTML from Assets without using WebViewAssetLoader using the following code, it doesn’t work.
web_view.loadUrl("file:///android_asset/index.html");
This is because a CORS error occurs when loading the JavaScript for the module.
Result
we are able to convert my Vite web app into an Android app.
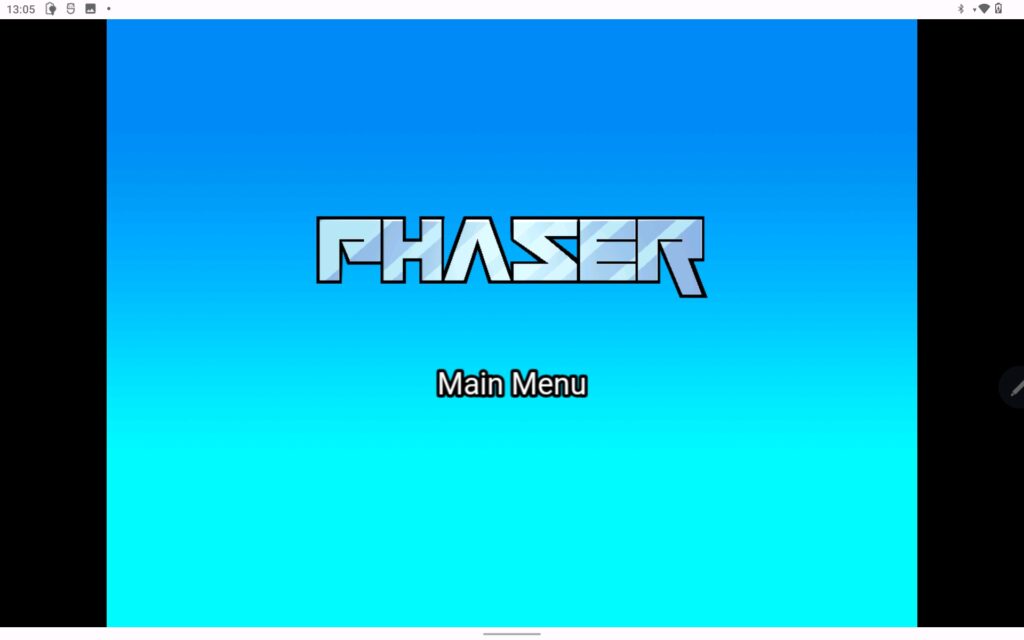
Reference
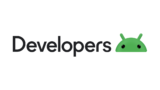
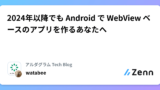
comment